Shift is a desktop app for streamlining all of your email and app accounts. Easily manage Gmail and Google Drive Messenger, WhatsApp, Slack, and 500+ apps.
So far, nearly all the articles I have seen about SwiftUI show it being used for iOS, more particularly for iPhone.But SwiftUI works on all Apple’s platforms, and as I am primarily a Mac developer, I decided to try out a Mac app and see what happened.
Swift Playgrounds is the latest of several of Apple's own apps to get the treatment. The Mac App will work in the same way as the iPad app, using the trackpad to navigate rather than a touchscreen. The Swift compiler and runtime are fully embedded throughout Xcode, so your app is constantly being built and run. The design canvas you see isn’t just an approximation of your user interface — it’s your live app. And Xcode can swap edited code directly in your live app with “dynamic replacement”, a new feature in Swift. Swift is a general-purpose, multi-paradigm, compiled programming language developed by Apple Inc. And the open-source community, first released in 2014.Swift was developed as a replacement for Apple's earlier programming language Objective-C, as Objective-C had been largely unchanged since the early 1980s and lacked modern language features.Swift works with Apple's Cocoa and Cocoa Touch.
Setup
I opened up Xcode and created a new project selecting the macOS App template. The project opened at the usual ContentView.swift but there were a few differences in the project structure as well as one in the ContentView struct.
The first thing to notice is that the default “Hello, World!” Text view has a frame set:
If I removed this frame modifier, the preview display in the Canvas changed so that the view was only the size of the text instead of being a standard window size. I guess an iOS device always knows what size it is, but a Mac window can be any size, so you have to be more explicit to stop SwiftUI shrinking the container view to the minimum size possible.
The next thing is to look at the files that are included in the project. There is no SceneDelegate.swift as you would see in an iOS project. And to my surprise, there was still a Main.storyboard file! And going to the General settings for the app target, I could see that this storyboard was selected as the Main Interface.
Opening it up reveals that this is where the application menu is configured. I had wondered where the menus were configured in Mac SwiftUI apps.
The AppDelegate was the next thing I looked at and here I found some of the code that I would have expected to find in a SceneDelegate. The applicationDidFinishLaunching(_:)
method creates an instance of ContentView, creates an NSWindow and uses an NSHostingView to display the ContentView inside the window. At this stage, running the app gives me what I would expect: a fully-fledged Mac app with a window and a menu, both with all the functions you would expect in any standard Mac app.
The Canvas
I was not expecting the Canvas to be much use when it came to previewing a Mac app. It works so well with an iPhone app because the iPhone is tall and thin and fits neatly into one side of the code window. But a Mac view is likely to be much bigger, so it would have to be scaled down a lot to avoid talking up too much precious space in my Xcode window.
But it works as expected, and even scaled down, you get a good idea of the layout while still getting the live reloading that is part of what makes developing in SwiftUI so much fun.
But here is where I got my first real surprise, with a feature that I had not seen yet in any SwiftUI tutorial or article. Click the Live Preview button and see what happens…
Of course I clicked “Bring Forward” and there was my app running in a window called “Xcode Preview”. There was an app in my Dock and when I chose “Show in Finder”, I found that the app is buried deep in DerivedData. Positioning my windows so I could type in Xcode while watching this preview window, I saw that it instantly updated my view as I typed, just like an iPhone in the Canvas.
If I changed the structure of the view, the app closed and re-opened immediately with the new content. This is amazing and shows that the Xcode & SwiftUI teams really thought about how to use these new features in Mac apps as well as iOS.
In Xcode 11.3, I found that I was having trouble with the previews. They would not display and above the Canvas, I got the super helpful message “Cannot preview in this file — SwiftUI-Mac.app may have crashed.”. It turned out that this was a signing issue. If you go to the app target and look in the Signing and Capabilities section, check that Signing Certificate is not set to “Sign to Run Locally”. If it is, switch to “Development” and the previews will start working again.
Laying out the View
Now that I have the project and I know how to preview it, it’s time to work out what to display in the app. The next real app I want to work on will use a master-detail layout, so that is what I decided to try here.
Before worrying about the data, I decided to try populating the master view with a static list and using that to navigate to a detail view that was simply a Text view.
This worked, except that the left column was only about 20 pixels wide. But I was able to use the mouse to drag it wider and there were my List entries. Clicking on one did indeed show the detail I wanted, but it shrunk the window to one line high!
The first thing I did was to apply a listStyle
modifier to make it show the semi-transparent Mac sidebar. This fixed the width of the sidebar. But the whole window still shrunk when I selected an item.
I tried applying the frame modifier to the NavigationView and that made the window stay the same size, but the content still shrunk into a tiny section in the middle. It looks like I need to apply that frame modifier to the detail view as well.
And as you can see from this gif, I then had a full functional master-detail view with a collapsible and expandable semi-transparent sidebar.
Adding Data
After some scouting around for a free API that I could hook into, I came across HTTP Cats which is a site that serves up a cat image to match almost every HTTP status code.
This sounded ideal: I can list the codes in the master view on the left and display the image in the detail view on the right.
First I created a JSON file to list all the HTTP status codes so that I could put them into a List view. This was a very simple array with each entry having a code and a title:
I created an HttpStatus struct with these 2 properties and I borrowed Paul Hudson’s excellent Helper Bundle extension to decode the JSON file. For a first attempt, I used the numeric codes to build the list and showed the title of the selected one in the detail view. But one of the best things about SwiftUI is that it makes it so easy to configure table rows, so it is time to create a new View to do this.
After some experimentation, I had a TableRowView that I liked the look of, but the default sidebar width was too narrow and truncated the status code titles, so I added a frame modifier to the List to set a minimum and maximum width for the sidebar.
Outline List
At this point I decided that it would be more useful to have a outline list with the status codes grouped by their category.
So I re-did the JSON file to show this, added an HttpSection struct and a SectionHeaderView and modified the data loading method and @State variable.
This worked really well and I was thrilled to find that the sections automatically had Show/Hide toggles!
Detail View
Up until now, I had been using a standard Text view as the destination for my navigation. This is a really useful technique as you can build the interface gradually but have it work from the beginning. But now it was time to create a new view for the details.
I set up the view and added a method that would download the correct cat image when the view appeared but there was no image. After some digging, I realised that sand-boxed Mac apps do not allow network access by default. I went to the Signing & Capabilities section of the target settings and turned on “Outgoing Connections (Client)”. And then I had my cat pictures.
It really should have a loading image to display while the cat image is being downloaded, but to my disappointment, I found that the SF Symbols are not available to a Mac app! But I added a Text view to say “Loading…”.
Now that I have a functioning Mac app with a Master-Detail view, the next thing is to explore some more of the challenges that will need to be solved before I can write a Mac app completely using SwiftUI.
If you want to check out the project at this stage, here is a link to the relevant GitHub commit. Or if you would prefer, here is a link to the final version of the project.
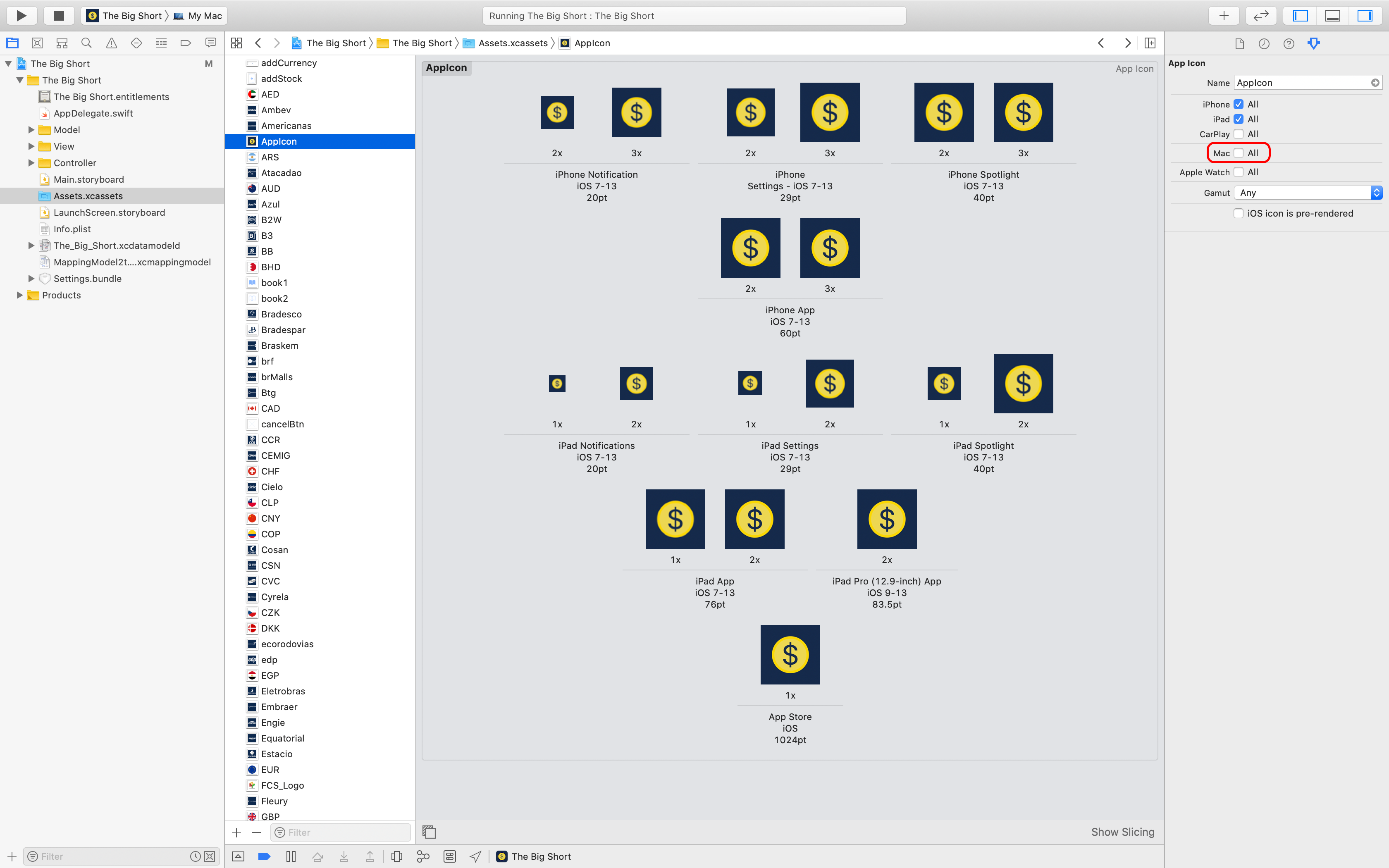
In part 2 of this series, I will look into:
- how to interact with the menus
- how to open a secondary window
- more user interface controls
- how to pass data around between windows
Here is the complete guide how to download Swift Wifi For PC laptop on Windows 10, 8.1, 8, 7, XP or Swift Wifi for MAC OS computers.
Swift Wifi – Global wifi sharing app offers you nearby WiFi options, which includes free WiFi and hotspots with passwords shared by our users all around the world, the availability will be automatically detected by Swift WiFi. Safety test function of our app is suggested to use before you get connected to a new WiFi, especially public WiFi and open WiFi to ensure your online safety. Swift WiFI is top 1 app in travel category.You may need to take pictures of your food and send “wish you were here!” tweets and Facebook updates, there is absolutely no reason to keep your roaming data on the entire time. Stay connected worldwide is easy with Swift WiFi since the app has a large and growing database with millions of users contribute to the sharing economy.
Except helping you find free WiFi, Swift WiFi is also an efficient WiFi management tool that improves WiFi performance, optimize WiFi connection by connecting to the optimal signal. Through Smart WiFi function, you can set specific mode to turn on/off WiFi under different circumstances so as to save battery and reduce radiation. Swift Wifi is available for Android mobiles. Swift Wifi for PC Windows or Swift Wifi for MAC is not available officially. There are many who wished to use Swift WiFi for PC Windows 10, 8.1, 8, 7, XP or Swift WiFi for MAC OS computer. For all those who wished to download Swift WiFi for PC today, here we are going to present the guide how to download Swift WiFi for PC Windows 10, 8.1, 8, 7, XP or Swift WiFi for MAC OS computers.
FEATURES OF SWIFT WIFI APP
Before going to the procedure to download Swift Wifi For PC laptop on Windows 10, 8.1, 8, 7, XP or Swift Wifi for MAC OS computers, let’s see the features of app.
- Scan for WiFi hotspots and search for free WiFi around you. If no results found, it will get directions that guide you to the nearest WiFi spot.
- Safety test: Avoid insecure public WiFi or fishing hotspots, protect your online security.
- Speed test: Accurate network speed test.
- Smart WiFi: Reduce phone radiation and save battery power.
- Fast Charge: Kill power draining apps to accelerate charging speed.
- Share Free WiFi: You can add Free WiFi spots by sharing the passwords with other users.
DOWNLOAD SWIFT WIFI FOR PC LAPTOP ON WINDOWS 10/8.1/8/7/XP OR SWIFT WIFI FOR MAC OS COMPUTER USING BLUESTACKS
We have to use emulators to get Swift WiFi for PC laptop running Windows or MAC OS computer. They are many best Android emulators available among which Blustacks app player is the one best. So, today we are going to use Bluestacks emulator for installing Swift WiFi for PC . Before going to the procedure first check the below guide to download Blustacks app player to your PC.
- Once you are done with the Bluestacks installation, launch the app on your Windows PC.
- Just click on the Search box in Bluestacks app.
- Now search Swift WiFi in the search box and click on Search Play for Swift WiFi.
- You will be redirected to Google Play store.Click on Swift WiFi app Icon.
- Now, click on Install button to start the installation of Swift WiFi for PC and wait till the installation completed.
- Once installation completed just navigate to Bluestacks Homepage > All Apps.
- There you can find Swift WiFi app installed on your PC. Launch the Swift WiFi on PC and enjoy the features of the app.
DOWNLOAD SWIFT WIFI FOR PC LAPTOP ON WINDOWS 10/8.1/8/7/XP OR SWIFT WIFI FOR MAC WITH APK FILE
If you have any error while installing Swift WiFi for PC using above method, below method to install.
- As a first step download bluestacks app player from the link provided in the above method if you have not downloaded yet.
- Download Swift WiFi APK file from below link.
- Once downloaded, right click on APK file and tap on Open with Bluestacks Player.

This initiates the installation of Swift WiFi for PC for you. Once installation completed navigate to bluestacks Homepage > All Apps where you can find Swift WiFi for PC laptop installed on your Windows or MAC OS computer.
CONCLUSION
That’s it the procedure to download Swift WiFi for PC laptop running Windows (10/8.1/8/7/XP) or MAC OS computer. Hope you have successfully installed the app. If you have any issues while installing the app, please let us know through comments so that our team will help you in resolving the issue. Do share it on Social media if it meant useful. Thanks for visiting us. Keep visiting us for more updates.
You can join us on Facebook or Twitter for keeping yourself updated with latest news and apps.
Swift For Mac App Free
Related
